Nodejs SDK Guide
We have written a step-by-step guide to help you build your own PFM app using Brick. The code is an example of an Express+ NodeJS app that allows you to link Financial Institution accounts through Brick's API using our widget. Once an account is linked, you can access detailed information about the Accounts, Transactions, and Owners.
Click here to go to the repository. You can access all the app code and reuse it for your application.
This program is running on NodeJS v 14.15.3. You would be required to install Express, ejs, request, cors, and nodemon.
Installation
npm install express
npm install ejs
npm i brick-node-sdk
npm install nodemon --save-dev
npm install request --save
npm install cors --save
Project Path
The project folder name in this sample is NodeJS-Demo, in this folder you can then you can initialize in command line.
npm init
Then once you have the initial configuration files installed, you can create a server file, in this project sample we will call it app.js and save it in NodeJS-Demo folder.
- Next, you can create a new folder in your root folder (NodeJS-Demo) and name it views which will contains the index.ejs, error.ejs and result.ejs, these 2 files are basically the HTML side.
- Finally, in the root folder you can create a public folder which will have css folder and js folder.
- In this sample project the css folder will contain the main.css file.
So, your project path should look like the following,
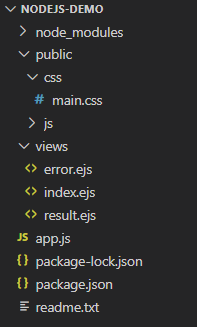
Usage
package.json
{
"name": "brick-demo",
"version": "1.0.0",
"description": "Brick NodeJS",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"start": "nodemon --no-deprecation app.js"//this start script will use nodemon with deprecation warning removed
},
"author": "",
"license": "ISC",
//you are required to have this dependeices
"dependencies": {
"cors": "^2.8.5",
"brick-node-sdk":"1.0.4",
"ejs": "^3.1.5",
"express": "^4.17.1",
"request": "^2.88.2"
},
"devDependencies": {
"nodemon": "^2.0.6"
}
}
Import Package
//import libraries
const express = require('express')//import express
const app = express()//app server to use express
const port = 3000//initialize port
const request = require('request')//import request
const cors = require('cors')//import cors
Server configuration
//access the json data from url in post or get, eg. to retrieve the user-access-token
app.use(express.json())
//access static file in the project folder
app.use(express.static('public'))
app.use('/css',express.static(__dirname + 'public/css'))
app.use(cors())//server to use cors function
//set view folder for ejs file
app.set('views','./views')
//set engine ejs so we dont need to use extension in string name
app.set('view engine','ejs')
Initializing Dictionary to store values
//dictionary for public-token and user-access-token
var dict = {
"public_token" : "",
"user_token" : ""
}
//dictionary for transactions detail
var transaction_dict = {
"date" : [],
"description":[],
"status" : [],
"amount" : []
}
//dictionary for user account list in one user ID
var user_account ={
"accountHolder" :[],
"accountNumber" :[],
"accountID":[],
"balance":{
"available" : "",
"current" : ""
}
}
//dicitonary for user details for specific user account
var user_detail ={
"accountHolder" :"",
"accountNumber" :"",
"accountID":"",
"balance":{
"available" : "",
"current" : ""
}
}
//boolean to check for Jenius Bank or not (MFA)
var boolean = {
"isJenius" : false
}
//dictionary to store user's Jenius Bank details & transactions
var jenius ={
"jeniusTransactionDetail" : [],
"jeniusAccountDetail" : []
}
base_link to access Brick API
//base link for BRICK API
var base_link = 'https://sandbox.onebrick.io/v1/'
Date variable to retrieve current date
//variable to get current date
var date = new Date()
var year = date.getFullYear()
var month = ("0" +date.getMonth()+1).slice(-2)
var day = ("0" +date.getDate()).slice(-2)
First Get function
This get function is in app.js server to initialize the index.ejs
//first function to be called when program is executed
app.get('/',(req,res)=>{
//render index.ejs
res.render('index')
})
index.ejs
This will be the starting HTML page when user execute the program.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel = "stylesheet" href = "css/main.css">
<title>Demo</title>
</head>
<body>
<div class="center-page">
<h2 class="app-name">Money Save App</h2>
<p class="detail">Welcome to Brick's PFM application. Please think of Money Save as your own application UI when using this demo.</p>
<div class="button-wrapper">
<form action ="accessbrick" method ="post" target="popup" onsubmit ="window.open('','popup','width=800, height=800');">
<input type="submit" value ="Add a Bank Account" style="margin:0px auto"></input>
</form>
</div>
</div>
</body>
</html>
POST method after index form is submitted
This POST method will redirect to Brick API once an authentication function is completed and successful.
//post function triggered when index form is submitted
app.post('/accessbrick', function(req,res){
//callback authentication function
authentication(function(body){
//redirect to BRICK API(call brick widget)
res.redirect(base_link+'index?'+"accessToken="+body+"&redirect_url="+"http://localhost:3000/result")
})
})
authentication function
This function will be called to authenticate the clientID and clientSecret before we use Brick API. This function will be called in app.post('/accessbrick')
// function to retrieve public access token
function authentication(callback){
//reset public token
dict["public_token"]=""
//variable for client ID and client secret
var clientID = "XXXXX"
var clientSecret = "XXXXX"
var account = clientID + ":" + clientSecret
var temp = new Buffer(account)
//buffer to convert client ID : client secret with base64
var authorize = "Basic "+ temp.toString('base64')
//headers for HTTP
var headers = {
'Content-type':'application/json',
'Authorization':authorize
}
//link parameters
var link = {
method : 'GET',
strictSSL : false,
url : base_link+"auth/token",
headers : headers
}
//request function
request(link, function (error, res, body){
//if not error
if(!error){
//retrieve public-access-token and store it to the dictionary['public_token']
var object = JSON.parse(body)
var public_token = object['data']['access_token']
dict["public_token"] = public_token
callback(public_token)
}else{
console.log(error)
}
})
}
Handling POST method from Brick Widget
This method is required in client's system to handle POST method from Brick Widget, such as retrieving user-access-token, bankId, or even Jenius Bank Transactions and Details. Also, this method will send the URL that will be redirected after Brick Widget UI.
//post function to BRICK WIDGET
app.post('/result', function(req,res){
//reset dictionaries value
dict["user_token"] = ""
jenius['jeniusTransactionDetail'] = []
jenius['jeniusAccountDetail'] = []
//variable to retrieve user_access_token
var object =req.body
//count the length of JSON response, if more than one then user is using Jenius Bank
var count = Object.keys(object).length;
//condition to check if user is using Jenius Bank or not
if(count > 2){
//when transactions is not null
if(object['transactions'] != null){
//set boolean if jenius bank is used
boolean['isJenius'] = true
//read the transaction response in JSON
var temp = JSON.parse(object['transactions'])
//iterate the tranasction list and push to an array
for (var i =0; i<temp.length;i++){
jenius['jeniusTransactionDetail'].push(temp[i])
}
}
if(object['accounts'] != null){
//read the transaction response in JSON
var temp2 = JSON.parse(object['accounts'])
//iterate the tranasction list and push to an array
for (var i =0; i<temp2.length;i++){
jenius['jeniusAccountDetail'].push(temp2[i])
}
}
}
//retrieve user-access-token from the POST response
var user_token = object['accessToken']
dict['user_token'] = user_token
//variable to generate sessionId
var sessionId =''
var chars ='0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'
for (var i = 20; i > 0; --i){
sessionId += chars[Math.floor(Math.random() * chars.length)]
}
//sent post value
res.send('http://localhost:3000/result?sessionId='+sessionId)
})
Handling GET method from Brick Widget
This method is required in client's system to handle GET method from Brick Widget, which will return the redirect URL and pass the values retrieved from Brick API.
//get function to BRICK WIDGET
app.get('/result', function(req,res){
//condition when user-access-token is available
if(dict['user_token'] != ""){
//condition when user is using Jenius Bank
if(boolean['isJenius'] == true){
//call getTransactionJenius to retrieve all the transaction details
getTransactionJenius(function(transaction_body){
getUserDetailJenius(function(detail_body){
var transaction = transaction_body
var userDetail = detail_body
//render result page and send over the transaction details and date
res.render('result', {userDetail:userDetail,transaction:transaction, date: year+'-'+month+'-'+day})
})
})
//user using other bank than Jenius Bank
}else{
//call getTransaction to retrieve all the transaction details
getTransaction(function(transaction_body){
//call getUserDetail to retrieve all user details
getUserDetail(function(detail_body){
//variable to store the function return values
var transaction = transaction_body
var userDetail = detail_body
//render result page and send over the transaction details, user details, and date
res.render('result', {userDetail:userDetail, transaction:transaction, date: year+'-'+month+'-'+day})
})
})
}
//condition when user-access-token is not available
}else{
res.render('error')
}
})
result.ejs
This will be the result(redireted) HTML page when user execute the program.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel = "stylesheet" href = "css/main.css">
<title>Transactions</title>
</head>
<body>
<div class="container">
<div class ="header">
<div class ="personal-detail">
<h2 class="title">Hello!</h2>
<h2 class="title-account">
<%= JSON.parse(JSON.stringify(userDetail['accountNumber']))%>
</h2>
<h2 class="title-date"><%= date%></h2>
</div>
</div>
<div class="balance">
<h2>Balance</h2>
<hr>
<div class="balance-wrapper">
<div class="available-balance">
<h2>Rp <%= JSON.parse(JSON.stringify(userDetail['balance']['available']))%></h2>
<p style="text-align:center">Available Balance</p>
</div>
<div class="current-balance">
<h2>Rp <%= JSON.parse(JSON.stringify(userDetail['balance']['current']))%></h2>
<p style="text-align:center">Current Balance</p>
</div>
</div>
<hr>
</div>
<div class="transaction">
<h2>Transaction</h2>
<hr>
<table class="transaction">
<div class="test" style="height:100%">
<tr>
<th class="time">Time</th>
<th class="description">Description</th>
<th class="status">Status</th>
<th class="amount">Amount</th>
</tr>
<% for(var i=0;i < transaction['description'].length; i++) { %>
<tr>
<td><%= JSON.parse(JSON.stringify(transaction['date'][i]))%></td>
<td><%= JSON.parse(JSON.stringify(transaction['description'][i]))%></td>
<td><%= JSON.parse(JSON.stringify(transaction['status'][i]))%></td>
<td>Rp<%= JSON.parse(JSON.stringify(transaction['amount'][i]))%></td>
</tr>
<% } %>
</div>
</table>
</div>
<div class="footer"></div>
</div>
</body>
</html>
getUserAccount function
In this function user will be able to retrieve all account list within one userID. This function will be called in getUserDetail function.
//function to get the account list
function getUserAccount(callback){
//reset user account dictionary
user_account['accountHolder'] = []
user_account['accountNumber'] = []
user_account['accountID'] = []
user_account['balance']['available'] = ""
user_account['balance']['current'] = ""
//get user token from dictionary which is retrieved from BRICK widget
var user_token = dict['user_token']
//variable headers for http
var headers = {
'Content-type':'application/json',
'Authorization':'Bearer ' + user_token
}
//link parameters
var link = {
method : 'GET',
strictSSL : false,
url : base_link+"account/list",
headers : headers
}
//request function
request(link, function (error, res, body){
if(!error){
//retrieve JSON reponse
var object = JSON.parse(body)
//store account list
var account_list = []
account_list.push(object['data'][0])
//if account list is empty, means we are using Mock Bank
if(account_list.length == 0){
user_account['accountHolder'].push("JohnDoe")
user_account['accountNumber'].push("9870675789")
user_account['accountID'].push("qwerty//++--==")
user_account['balance']['available']="100,987"
user_account['balance']['current']="100,987"
}
//else if account list exist
else{
//iterate through all the account list and store into user_account dictionary
for (var i = 0; i < account_list.length;i++){
user_account['accountHolder'].push(account_list[i]['accountHolder'])
user_account['accountNumber'].push(account_list[i]['accountNumber'])
user_account['accountID'].push(account_list[i]['accountId'])
user_account['balance']['available']=account_list[i]['balances']['available'].toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",")
user_account['balance']['current']=account_list[i]['balances']['current'].toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",")
}
}
//callback the user_account dictionary
callback(user_account)
}else{
console.log(error)
}
})
}
Example of Account list in JSON format that we retrieved
{
"status": 200,
"message": "OK",
"data": [
{
"accountId": "UarXg4ad/81344wJF8xthY==",
"accountHolder": "JOHN DOE",
"accountNumber": "1551110757891",
"balances": {
"available":22130.0,
"current": 22130.0
}
},
{
"accountId": "ZUTBeQcl2FTqNUwcVDuRFF==",
"accountHolder": "JOHN DOE",
"accountNumber": "1234567890123",
"balances": {
"available": 320554.15,
"current": 320554.15
}
}
]
}
getUserDetail function
This function will require an accountID to retrieve the details of that account, such as accountHolder, accountNumber, balances. This function will then be called in GET method to parse the values to result.ejs
//function to get account details
function getUserDetail(callback){
//reset user_detail in dictionary
user_detail['accountHolder'] = ""
user_detail['accountNumber'] = ""
user_detail['accountID'] = ""
user_detail['balance']['available'] = ""
user_detail['balance']['current'] = ""
//get the user-access-token from dictionary
var user_token = dict['user_token']
//call getUserAccount function to get the accountID
getUserAccount(function(account_body){
//variable headers for HTTP
var headers = {
'Content-type':'application/json',
'Authorization':'Bearer ' + user_token
}
//variable for accountID
var accountID = account_body['accountID']
//link parameter
var link = {
method : 'GET',
strictSSL : false,
url : base_link+"account/detail?accountId="+accountID,
headers : headers
}
//request function
request(link, function (error, res, body){
if(!error){
//variable to store json response
var object = JSON.parse(body)
var account_detail = object['data']
//populate user_detail dictionary with json reponses
user_detail['accountHolder'] = account_detail['accountHolder']
user_detail['accountNumber'] = account_detail['accountNumber']
user_detail['accountID'] = account_detail['accountId']
user_detail['balance']['available']=account_detail['balances']['available'].toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",")
user_detail['balance']['current']=account_detail['balances']['current'].toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",")
//return the dictionary
callback(user_detail)
}else{
console.log(error)
}
})
})
}
Example of Account details in JSON format that we retrieved
{
"status": "status",
"message": "message",
"data": {
"updateTimestamp": "2020-08-21T17:33:30",
"accountId": "jwYhjuy4tQZx8sZj3oBrg9fSqvzsR41F",
"accountHolder": "Jane Doe",
"accountNumber": "1342006123456",
"balances": {
"available": "675000.00",
"current": "675000.00"
},
"lastUpdated": "2020-08-31 14:27:37 +07:00",
"lastUpdatedTransaction": "2020-08-31 13:08:05 +07:00"
}
}
getTransaction function
This function will retrieve the transaction list from an account using date from and to as a parameter.
This function will be called in GET method to parse the value to result.ejs
//function to retrieve transactions
function getTransaction(callback){
//reset the transaction dictionary
transaction_dict['date']=[]
transaction_dict['description']=[]
transaction_dict['status']=[]
transaction_dict['amount']=[]
//get the user-access-token
var user_token = dict['user_token']
//variable headers for HTTP
var headers = {
'Content-type':'application/json',
'Authorization':'Bearer ' + user_token
}
//link parameter
var link = {
method : 'GET',
strictSSL : false,
url : base_link+"transaction/list?from=YYYY-MM-DD&to=YYYY-MM-DD",
headers : headers
}
//request function
request(link, function (error, res, body){
if(!error){
//variable to store JSON response
var object = JSON.parse(body)
var tran_list = object['data']
//populate transaction dictionary with JSON response
for (var i = 0; i < tran_list.length;i++){
transaction_dict['date'].push(tran_list[i]['date'])
transaction_dict['description'].push(tran_list[i]['description'])
transaction_dict['status'].push(tran_list[i]['status'])
transaction_dict['amount'].push(tran_list[i]['amount'].replace(/\B(?=(\d{3})+(?!\d))/g, ","))
}
//return transaction dictionary
callback(transaction_dict)
}else{
console.log(error)
}
})
}
Example of Account Transactions in JSON format that we retrieved
{
"status": 200,
"message": "OK",
"data": [
{
"id": 9744,
"account_id": "jwYhjuy4tQZx8sZj3oBrg9fSqvzsR41F==",
"category_id": 24,
"subcategory_id": 141,
"merchant_id": 8,
"location_country_id": 0,
"location_city_id": 0,
"outlet_outlet_id": 0,
"amount": 600000.0,
"date": "2020-06-29",
"description": "ATM-MP SA CWD XMD S1AW1MJK /7774759936/ATM-RCOASIAAFRK 4616993200225278 RCOASIAAFRK",
"status": "CONFIRMED",
"direction": "out"
},
{
"id": 9743,
"account_id": "jwYhjuy4tQZx8sZj3oBrg9fSqvzsR41F==",
"category_id": 22,
"subcategory_id": 129,
"merchant_id": 0,
"location_country_id": 0,
"location_city_id": 0,
"outlet_outlet_id": 0,
"amount": 1000.0,
"date": "2020-06-29",
"description": "CA/SA UBP DR/CR-ATM UBP60116073701FFFFFF085755130021 50000 S1AW1MJK /7774759939/ATM-RCOASIAAFRK P085755130021",
"status": "CONFIRMED",
"direction": "out"
}
]
}
getTransactionJenius function
This function will retrieve a user's account transaction if they use Jenius Bank. This function will be called in GET method to parse the value to result.ejs.
//function get Transaction if user uses Jenius Bank
function getTransactionJenius(callback){
//reset transasction dictionary
transaction_dict['date']=[]
transaction_dict['description']=[]
transaction_dict['status']=[]
transaction_dict['amount']=[]
//populate transaction dictionary with response from POST method in Brick API
for (var i = 0; i < jenius['jeniusTransactionDetail'].length;i++){
transaction_dict['date'].push(jenius['jeniusTransactionDetail'][i]['date'])
transaction_dict['description'].push(jenius['jeniusTransactionDetail'][i]['description'])
transaction_dict['status'].push(jenius['jeniusTransactionDetail'][i]['status'])
transaction_dict['amount'].push(jenius['jeniusTransactionDetail'][i]['amount'])
}
//return transaction dictionary
callback(transaction_dict)
}
getUserDetail function
This function will retrieve a user's account detail if they use Jenius Bank. This function will be called in GET method to parse the value to result.ejs.
//function getUserDetail if user uses Jenius Bank
function getUserDetailJenius(callback){
//reset user_detail dictionary
user_detail['accountHolder'] = ""
user_detail['accountNumber'] = ""
user_detail['accountID'] = ""
user_detail['balance']['available'] = ""
user_detail['balance']['current'] = ""
//populate user_detail dictionary with response from POST method in Brick API
for (var i = 0; i < jenius['jeniusAccountDetail'].length;i++){
user_detail['accountHolder'] = jenius['jeniusAccountDetail'][0]['accountHolder']
user_detail['accountNumber'] = jenius['jeniusAccountDetail'][0]['accountNumber']
user_detail['accountID'] = jenius['jeniusAccountDetail'][0]['accountId']
user_detail['balance']['available']= (jenius['jeniusAccountDetail'][0]['balances']['available']).toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",")
user_detail['balance']['current']= (jenius['jeniusAccountDetail'][0]['balances']['current']).toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",")
}
//return user_detail dictionary
callback(user_detail)
}
app.js
Your final code should look like the following.
//import libraries
const express = require('express')
const app = express()
const port = 3000
const request = require('request')
const cors = require('cors')
//access the json data from url in post or get, eg. to retrieve the user-access-token
app.use(express.json())
//access static file
app.use(express.static('public'))
app.use('/css',express.static(__dirname + 'public/css'))
app.use(cors())
// app.use('/js',express.static(__dirname + 'public/js'))
//set view folder for ejs file
app.set('views','./views')
//set engine ejs so we dont need to use extension in string name
app.set('view engine','ejs')
//dictionary for public-token and user-access-token
var dict = {
"public_token" : "",
"user_token" : ""
}
//dictionary for transactions detail
var transaction_dict = {
"date" : [],
"description":[],
"status" : [],
"amount" : []
}
//dictionary for user account list in one user ID
var user_account ={
"accountHolder" :[],
"accountNumber" :[],
"accountID":[],
"balance":{
"available" : "",
"current" : ""
}
}
//dicitonary for user details for specific user account
var user_detail ={
"accountHolder" :"",
"accountNumber" :"",
"accountID":"",
"balance":{
"available" : "",
"current" : ""
}
}
//boolean to check for Jenius Bank or not (MFA)
var boolean = {
"isJenius" : false
}
//boolean to store jenius bank details
var jenius ={
"jeniusTransactionDetail" : [],
"jeniusAccountDetail" : []
}
//base link for BRICK API
var base_link = 'https://sandbox.onebrick.io/v1/'
//variable to get current date
var date = new Date()
var year = date.getFullYear()
var month = ("0" +date.getMonth()+1).slice(-2)
var day = ("0" +date.getDate()).slice(-2)
//first function to be called when program is executed
app.get('/',(req,res)=>{
//render index.ejs
res.render('index')
})
//post function triggered when index form is submitted
app.post('/accessbrick', function(req,res){
//callback authentication function
authentication(function(body){
//redirect to BRICK API(call brick widget)
res.redirect(base_link+'index?'+"accessToken="+body+"&redirect_url="+"http://localhost:3000/result")
})
})
//post function to BRICK WIDGET
app.post('/result', function(req,res){
//reset dictionaries value
dict["user_token"] = ""
jenius['jeniusTransactionDetail'] = []
jenius['jeniusAccountDetail'] = []
//variable to retrieve user_access_token
var object =req.body
//count the length of JSON response, if more than one then user is using Jenius Bank
var count = Object.keys(object).length;
//condition to check if user is using Jenius Bank or not
if(count > 2){
//when transactions is not null
if(object['transactions'] != null){
//set boolean if jenius bank is used
boolean['isJenius'] = true
//read the transaction response in JSON
var temp = JSON.parse(object['transactions'])
//iterate the tranasction list and push to an array
for (var i =0; i<temp.length;i++){
jenius['jeniusTransactionDetail'].push(temp[i])
}
}
if(object['accounts'] != null){
//read the transaction response in JSON
var temp2 = JSON.parse(object['accounts'])
//iterate the tranasction list and push to an array
for (var i =0; i<temp2.length;i++){
jenius['jeniusAccountDetail'].push(temp2[i])
}
}
}
//retrieve user-access-token from the POST response
var user_token = object['accessToken']
dict['user_token'] = user_token
//variable to generate sessionId
var sessionId =''
var chars ='0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'
for (var i = 20; i > 0; --i){
sessionId += chars[Math.floor(Math.random() * chars.length)]
}
//sent post value
res.send('http://localhost:3000/result?sessionId='+sessionId)
})
//get function to BRICK WIDGET
app.get('/result', function(req,res){
//condition when user-access-token is available
if(dict['user_token'] != ""){
//condition when user is using Jenius Bank
if(boolean['isJenius'] == true){
//call getTransactionJenius to retrieve all the transaction details
getTransactionJenius(function(transaction_body){
getUserDetailJenius(function(detail_body){
var transaction = transaction_body
var userDetail = detail_body
//render result page and send over the transaction details and date
res.render('result', {userDetail:userDetail,transaction:transaction, date: year+'-'+month+'-'+day})
})
})
//user using other bank than Jenius Bank
}else{
//call getTransaction to retrieve all the transaction details
getTransaction(function(transaction_body){
//call getUserDetail to retrieve all user details
getUserDetail(function(detail_body){
//variable to store the function return values
var transaction = transaction_body
var userDetail = detail_body
//render result page and send over the transaction details, user details, and date
res.render('result', {userDetail:userDetail, transaction:transaction, date: year+'-'+month+'-'+day})
})
})
}
//condition when user-access-token is not available
}else{
res.render('error')
}
})
// function to retrieve public access token
function authentication(callback){
//reset public token
dict["public_token"]=""
//variable for client ID and client secret
var clientID = "XXXXX"
var clientSecret = "XXXXX"
var account = clientID + ":" + clientSecret
var temp = new Buffer(account)
//buffer to convert client ID : client secret with base64
var authorize = "Basic "+ temp.toString('base64')
//headers for HTTP
var headers = {
'Content-type':'application/json',
'Authorization':authorize
}
//link parameters
var link = {
method : 'GET',
strictSSL : false,
url : base_link+"auth/token",
headers : headers
}
//request function
request(link, function (error, res, body){
//if not error
if(!error){
//retrieve public-access-token and store it to the dictionary['public_token']
var object = JSON.parse(body)
var public_token = object['data']['access_token']
dict["public_token"] = public_token
callback(public_token)
}else{
console.log(error)
}
})
}
//function to get the account list
function getUserAccount(callback){
//reset user account dictionary
user_account['accountHolder'] = []
user_account['accountNumber'] = []
user_account['accountID'] = []
user_account['balance']['available'] = ""
user_account['balance']['current'] = ""
//get user token from dictionary which is retrieved from BRICK widget
var user_token = dict['user_token']
//variable headers for http
var headers = {
'Content-type':'application/json',
'Authorization':'Bearer ' + user_token
}
//link parameters
var link = {
method : 'GET',
strictSSL : false,
url : base_link+"account/list",
headers : headers
}
//request function
request(link, function (error, res, body){
if(!error){
//retrieve JSON reponse
var object = JSON.parse(body)
//store account list
var account_list = []
account_list.push(object['data'][0])
//if account list is empty, means we are using Mock Bank
if(account_list.length == 0){
user_account['accountHolder'].push("JohnDoe")
user_account['accountNumber'].push("9870675789")
user_account['accountID'].push("qwerty//++--==")
user_account['balance']['available']="100,987"
user_account['balance']['current']="100,987"
}
//else if account list exist
else{
//iterate through all the account list and store into user_account dictionary
for (var i = 0; i < account_list.length;i++){
user_account['accountHolder'].push(account_list[i]['accountHolder'])
user_account['accountNumber'].push(account_list[i]['accountNumber'])
user_account['accountID'].push(account_list[i]['accountId'])
user_account['balance']['available']=account_list[i]['balances']['available'].toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",")
user_account['balance']['current']=account_list[i]['balances']['current'].toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",")
}
}
//callback the user_account dictionary
callback(user_account)
}else{
console.log(error)
}
})
}
//function to get account details
function getUserDetail(callback){
//reset user_detail in dictionary
user_detail['accountHolder'] = ""
user_detail['accountNumber'] = ""
user_detail['accountID'] = ""
user_detail['balance']['available'] = ""
user_detail['balance']['current'] = ""
//get the user-access-token from dictionary
var user_token = dict['user_token']
//call getUserAccount function to get the accountID
getUserAccount(function(account_body){
//variable headers for HTTP
var headers = {
'Content-type':'application/json',
'Authorization':'Bearer ' + user_token
}
//variable for accountID
var accountID = account_body['accountID']
//link parameter
var link = {
method : 'GET',
strictSSL : false,
url : base_link+"account/detail?accountId="+accountID,
headers : headers
}
//request function
request(link, function (error, res, body){
if(!error){
//variable to store json response
var object = JSON.parse(body)
var account_detail = object['data']
//populate user_detail dictionary with json reponses
user_detail['accountHolder'] = account_detail['accountHolder']
user_detail['accountNumber'] = account_detail['accountNumber']
user_detail['accountID'] = account_detail['accountId']
user_detail['balance']['available']=account_detail['balances']['available'].toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",")
user_detail['balance']['current']=account_detail['balances']['current'].toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",")
//return the dictionary
callback(user_detail)
}else{
console.log(error)
}
})
})
}
//function to retrieve transactions
function getTransaction(callback){
//reset the transaction dictionary
transaction_dict['date']=[]
transaction_dict['description']=[]
transaction_dict['status']=[]
transaction_dict['amount']=[]
//get the user-access-token
var user_token = dict['user_token']
//variable headers for HTTP
var headers = {
'Content-type':'application/json',
'Authorization':'Bearer ' + user_token
}
//link parameter
var link = {
method : 'GET',
strictSSL : false,
url : base_link+"transaction/list?from=YYYY-MM-DD&to=YYYY-MM-DD",
headers : headers
}
//request function
request(link, function (error, res, body){
if(!error){
//variable to store JSON response
var object = JSON.parse(body)
var tran_list = object['data']
//populate transaction dictionary with JSON response
for (var i = 0; i < tran_list.length;i++){
transaction_dict['date'].push(tran_list[i]['date'])
transaction_dict['description'].push(tran_list[i]['description'])
transaction_dict['status'].push(tran_list[i]['status'])
transaction_dict['amount'].push(tran_list[i]['amount'].replace(/\B(?=(\d{3})+(?!\d))/g, ","))
}
//return transaction dictionary
callback(transaction_dict)
}else{
console.log(error)
}
})
}
//function get Transaction if user uses Jenius Bank
function getTransactionJenius(callback){
//reset transasction dictionary
transaction_dict['date']=[]
transaction_dict['description']=[]
transaction_dict['status']=[]
transaction_dict['amount']=[]
//populate transaction dictionary with response from POST method in Brick API
for (var i = 0; i < jenius['jeniusTransactionDetail'].length;i++){
transaction_dict['date'].push(jenius['jeniusTransactionDetail'][i]['date'])
transaction_dict['description'].push(jenius['jeniusTransactionDetail'][i]['description'])
transaction_dict['status'].push(jenius['jeniusTransactionDetail'][i]['status'])
transaction_dict['amount'].push(jenius['jeniusTransactionDetail'][i]['amount'])
}
//return transaction dictionary
callback(transaction_dict)
}
//function getUserDetail if user uses Jenius Bank
function getUserDetailJenius(callback){
//reset user_detail dictionary
user_detail['accountHolder'] = ""
user_detail['accountNumber'] = ""
user_detail['accountID'] = ""
user_detail['balance']['available'] = ""
user_detail['balance']['current'] = ""
//populate user_detail dictionary with response from POST method in Brick API
for (var i = 0; i < jenius['jeniusAccountDetail'].length;i++){
user_detail['accountHolder'] = jenius['jeniusAccountDetail'][0]['accountHolder']
user_detail['accountNumber'] = jenius['jeniusAccountDetail'][0]['accountNumber']
user_detail['accountID'] = jenius['jeniusAccountDetail'][0]['accountId']
user_detail['balance']['available']= (jenius['jeniusAccountDetail'][0]['balances']['available']).toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",")
user_detail['balance']['current']= (jenius['jeniusAccountDetail'][0]['balances']['current']).toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",")
}
//return user_detail dictionary
callback(user_detail)
}
//function listen to port
app.listen(port, function(){
console.info(`Listening to port ${port}`)
})
Updated over 2 years ago